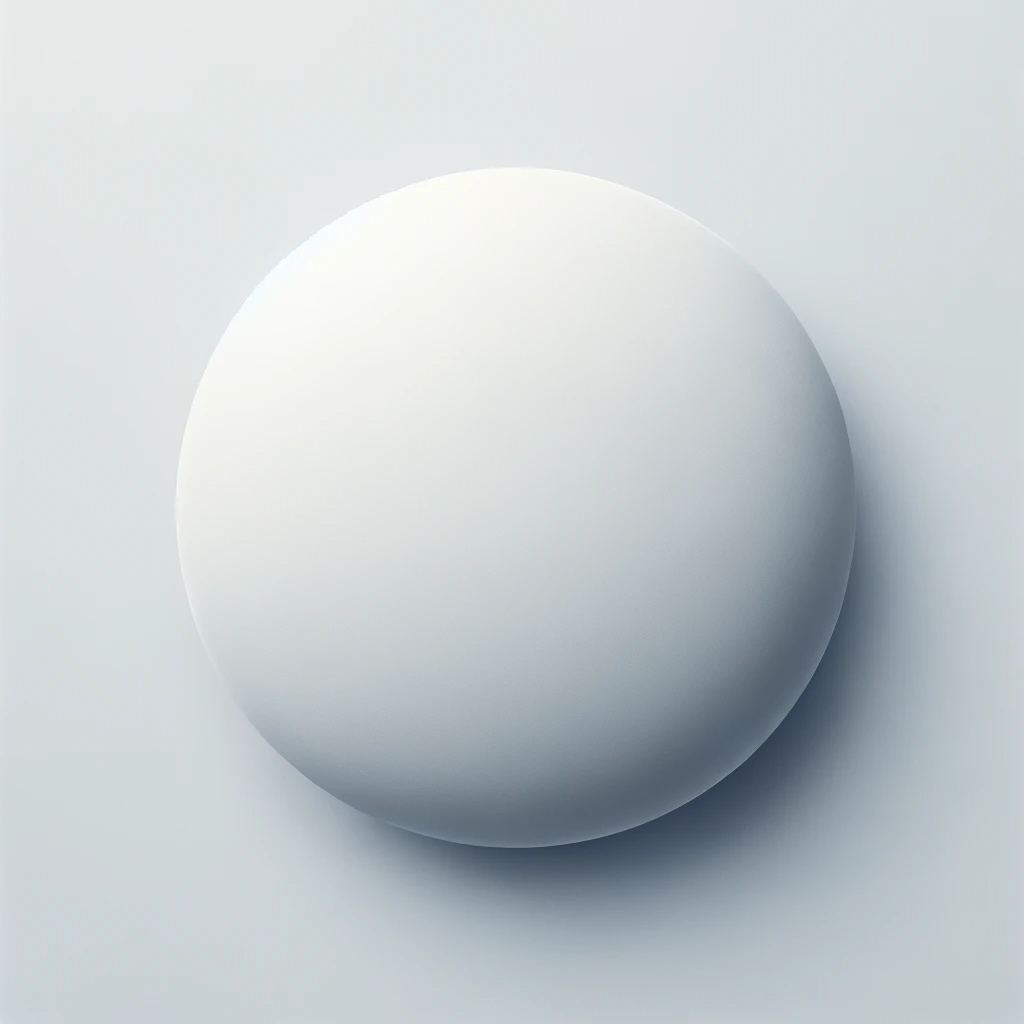
PyGAD - Python Genetic Algorithm!¶ PyGAD is an open-source Python library for building the genetic algorithm and optimizing machine learning algorithms. It works with Keras and PyTorch. PyGAD supports different types of crossover, mutation, and parent selection operators. PyGAD allows different types of problems to be optimized using the genetic …Sep 28, 2021 ... scipy.optimize.minimize can also handle some kinds of constraints. We examine how to minimize a function in Python where there are equality ...Performance options ¶. Configuring Python using --enable-optimizations --with-lto (PGO + LTO) is recommended for best performance. The experimental --enable-bolt flag can also be used to improve performance. Enable Profile Guided Optimization (PGO) using PROFILE_TASK (disabled by default).Multiple variables in SciPy's optimize.minimize. According to the SciPy documentation, it is possible to minimize functions with multiple variables, yet it doesn't say how to optimize such functions. return sqrt((sin(pi/2) + sin(0) + sin(c) - 2)**2 + (cos(pi/2) + cos(0) + cos(c) - 1)**2) The above code try to minimize the function f, but for my ...The following is a toy example (evidently this one could be solved using the gradient): # import minimize from scipy.optimize import minimize # define a toy function to minimize def my_small_func(g): x = g[0] y = g[1] return x**2 - 2*y + 1 # define the starting guess start_guess = [.5,.5] # define the acceptable ranges (for [g1, g2] repectively) …scipy.optimize.curve_fit # scipy.optimize.curve_fit(f, xdata, ydata, p0=None, sigma=None, absolute_sigma=False, check_finite=None, bounds=(-inf, inf), …Python is a powerful and versatile programming language that has gained immense popularity in recent years. Known for its simplicity and readability, Python has become a go-to choi...every optimization algorithm within scipy, will at most guarantee a local-optimum, which might be arbitrarily bad compared to the global-optimum; Assumption: M is positive-definite / negative-definite. If we assume matrix M is either positive-definite or negative-definite, but not indefinite, this is a convex-optimization problem.Sep 27, 2021 ... A common task in engineering is finding the minimum of a function. In this example, we show how to find a minimum graphically, ...SHGO stands for “simplicial homology global optimization”. The objective function to be minimized. Must be in the form f (x, *args), where x is the argument in the form of a 1-D array and args is a tuple of any additional fixed parameters needed to completely specify the function. Bounds for variables.Sourcery is a static code analysis tool for Python. It uses advanced algorithms to detect and correct common issues in your code, such as typos, formatting errors, and incorrect variable names. Sourcery also offers automated refactoring tools that help you optimize your code for readability and performance.This leads to AVC denial records in the logs. 2. If the system administrator runs python -OO [APP] the .pyos will get created with no docstrings. Some programs require docstrings in order to function. On subsequent runs with python -O [APP] python will use the cached .pyos even though a different …Hyperopt is a Python implementation of Bayesian Optimization. Throughout this article we’re going to use it as our implementation tool for executing these methods. I highly recommend this library! Hyperopt requires a few pieces of input in order to function: An objective function. A Parameter search space.Aug 25, 2022 · This leads to AVC denial records in the logs. 2. If the system administrator runs python -OO [APP] the .pyos will get created with no docstrings. Some programs require docstrings in order to function. On subsequent runs with python -O [APP] python will use the cached .pyos even though a different optimization level has been requested. Python is a versatile programming language that is widely used for game development. One of the most popular games created using Python is the classic Snake Game. To achieve optima...Jul 23, 2021 · The notebook illustrates one way of doing this, called a points race. Using HumpDay points_race to assess optimizer performance on a list of objective functions. Maybe that takes too long for your ... Bayesian Optimization of Hyperparameters with Python. Choosing a good set of hyperparameters is one of most important steps, but it is annoying and time consuming. The small number of hyperparameters may allow you to find an optimal set of hyperparameters after a few trials. This is, however, not the case for complex models like …Python equivalence to inline functions or macros. where x is a numpy array of complex numbers. For code readability, I could define a function like. return x.real*x.real+x.imag*x.imag. which is still far faster than abs (x)**2, but it is at the cost of a function call.Optimization is the problem of finding a set of inputs to an objective function that results in a maximum or minimum function evaluation. It is the challenging problem that underlies many machine learning algorithms, from fitting logistic regression models to training artificial neural networks. There are perhaps hundreds of popular optimization …Bayesian Optimization of Hyperparameters with Python. Choosing a good set of hyperparameters is one of most important steps, but it is annoying and time consuming. The small number of hyperparameters may allow you to find an optimal set of hyperparameters after a few trials. This is, however, not the case for complex models like …Running A Portfolio Optimization. The two key inputs to a portfolio optimization are: Expected returns for each asset being considered.; The covariance matrix of asset returns.Embedded in this are information on cross-asset correlations and each asset’s volatility (the diagonals).; Expected returns are hard to estimate — some people …Dec 14, 2020 ... This book describes a tool for mathematical modeling: the Python Optimization. Modeling Objects (Pyomo) software.And run the optimization: results = skopt.forest_minimize(objective, SPACE, **HPO_PARAMS) That’s it. All the information you need, like the best parameters or scores for each iteration, are kept in the results object. Go here for an example of a full script with some additional bells and whistles.SciPy is a Python library that is available for free and open source and is used for technical and scientific computing. It is a set of useful functions and mathematical methods created using Python’s NumPy module. ... Import the optimize.linprog module using the following command. Create an array of the …An optimizer is one of the two arguments required for compiling a Keras model: You can either instantiate an optimizer before passing it to model.compile () , as in the above example, or you can pass it by its string identifier. In the latter case, the default parameters for the optimizer will be used.Moment Optimization introduces the momentum vector.This vector is used to “store” changes in previous gradients. This vector helps accelerate stochastic gradient descent in the relevant direction and dampens oscillations. At each gradient step, the local gradient is added to the momentum vector. Then parameters are updated just by …Dec 31, 2016 · 1 Answer. Sorted by: 90. This flag enables Profile guided optimization (PGO) and Link Time Optimization (LTO). Both are expensive optimizations that slow down the build process but yield a significant speed boost (around 10-20% from what I remember reading). The discussion of what these exactly do is beyond my knowledge and probably too broad ... Replace the code from the editor above with the following 3 lines of code to see the output: numbers = pd.DataFrame ( [2,3,-5,3,-8,-2,7]) numbers ['Cumulative Sum'] = numbers.cumsum () numbers. This case becomes really useful in optimization tasks such as this Python optimization question and whenever we need to analyse a number that …Replace the code from the editor above with the following 3 lines of code to see the output: numbers = pd.DataFrame ( [2,3,-5,3,-8,-2,7]) numbers ['Cumulative Sum'] = numbers.cumsum () numbers. This case becomes really useful in optimization tasks such as this Python optimization question and whenever we need to analyse a number that …Python and Scipy Optimization implementation. 1. Improving the execution time of matrix calculations in Python. 1. Runtime Optimization of sympy code using numpy or scipy. 4. Optimization in scipy from sympy. 3. Code optimization python. 2. Speeding up numpy small function. Hot Network QuestionsThe codon optimization models for Escherichia Coli were trained by the Bidirectional Long-Short-Term Memory Conditional Random Field. Theoretically, deep learning is a good method to obtain the ...Here are three strategies to accelerate your Generative AI rollout: Partner with SaaS Leaders who have already mastered the art of building …We remark that not all optimization methods support bounds and/or constraints. Additional information can be found in the package documentation. 3. Conclusions. In this post, we explored different types of optimization constraints. In particular, we shared practical Python examples using the SciPy library. The …Description. Mathematical Optimization is getting more and more popular in most quantitative disciplines, such as engineering, management, economics, and operations research. Furthermore, Python is one of the most famous programming languages that is getting more attention nowadays. Therefore, we decided to …From a mathematical foundation viewpoint, it can be said that the three pillars for data science that we need to understand quite well are Linear Algebra, Statistics and the third pillar is Optimization which is used pretty much in all data science algorithms. And to understand the optimization concepts one needs a good fundamental understanding of … Bayesian optimization works by constructing a posterior distribution of functions (gaussian process) that best describes the function you want to optimize. As the number of observations grows, the posterior distribution improves, and the algorithm becomes more certain of which regions in parameter space are worth exploring and which are not, as ... Optimization in SciPy. Optimization seeks to find the best (optimal) value of some function subject to constraints. \begin {equation} \mathop {\mathsf {minimize}}_x f (x)\ \text {subject to } c (x) \le b \end {equation} import numpy as np import scipy.linalg as la import matplotlib.pyplot as plt import scipy.optimize as opt.May 25, 2022 · Newton’s method for optimization is a particular case of a descent method. With “ f′′ (xk ) ” being the derivative of the derivative of “ f” evaluated at iteration “ k”. Consider ... By Adrian Tam on October 30, 2021 in Optimization 45. Optimization for Machine Learning Crash Course. Find function optima with Python in 7 days. All machine learning models involve optimization. As a practitioner, we optimize for the most suitable hyperparameters or the subset of features. Decision tree algorithm …Python is one of the most popular programming languages in the world. It is known for its simplicity and readability, making it an excellent choice for beginners who are eager to l...Bayesian optimization is a machine learning based optimization algorithm used to find the parameters that globally optimizes a given black box function. There are 2 important components within this algorithm: The black box function to optimize: f ( x ). We want to find the value of x which globally optimizes f ( x ).From a mathematical foundation viewpoint, it can be said that the three pillars for data science that we need to understand quite well are Linear Algebra, Statistics and the third pillar is Optimization which is used pretty much in all data science algorithms. And to understand the optimization concepts one needs a good fundamental understanding of …Visualization for Function Optimization in Python. By Jason Brownlee on October 12, 2021 in Optimization 5. Function optimization involves finding the input that results in the optimal value from an objective function. Optimization algorithms navigate the search space of input variables in order to locate the optima, and both the shape of the ...Dec 17, 2021 · An Introduction to Numerical Optimization with Python (Part 1) 13 minute read. Published:December 17, 2021. This is the first post in a series of posts that I am planning to write on the topic of machine learning. This article introduces fundamental algorithms in numerical optimization. For now, this is the Gradient Descent and Netwon algorithm. Chapter 9 : Numerical Optimization. 9.1. Finding the root of a mathematical function *. 9.2. Minimizing a mathematical function. 9.3. Fitting a function to data with nonlinear least squares. 9.4. Finding the equilibrium state of a physical system by minimizing its potential energy. Aug 30, 2023 · 4. Hyperopt. Hyperopt is one of the most popular hyperparameter tuning packages available. Hyperopt allows the user to describe a search space in which the user expects the best results allowing the algorithms in hyperopt to search more efficiently. Currently, three algorithms are implemented in hyperopt. Random Search. Oct 12, 2021 · Optimization refers to a procedure for finding the input parameters or arguments to a function that result in the minimum or maximum output of the function. The most common type of optimization problems encountered in machine learning are continuous function optimization, where the input arguments to the function are real-valued numeric values ... 10000000 loops, best of 3: 0.0734 usec per loop. $ python -mtimeit -s'x=1' 'd=2' 'if x: d=1'. 10000000 loops, best of 3: 0.101 usec per loop. so you see: the "just-if" form can save 1.4 nanoseconds when x is false, but costs 40.2 nanoseconds when x is true, compared with the "if/else" form; so, in a micro-optimization context, you should use ...Modern society is built on the use of computers, and programming languages are what make any computer tick. One such language is Python. It’s a high-level, open-source and general-...The Nelder-Mead optimization algorithm can be used in Python via the minimize () function. This function requires that the “ method ” argument be set to “ nelder-mead ” to use the Nelder-Mead algorithm. It takes the objective function to be minimized and an initial point for the search. 1. 2. sys.flags.optimize gets set to 1. __debug__ is False. asserts don't get executed. In addition -OO has the following effect: sys.flags.optimize gets set to 2. doc strings are not available. To verify the effect for a different release of CPython, grep the source code for Py_OptimizeFlag. Python is a powerful and versatile programming language that has gained immense popularity in recent years. Known for its simplicity and readability, Python has become a go-to choi... Optimization Loop¶ Once we set our hyperparameters, we can then train and optimize our model with an optimization loop. Each iteration of the optimization loop is called an epoch. Each epoch consists of two main parts: The Train Loop - iterate over the training dataset and try to converge to optimal parameters. cvxpylayers. cvxpylayers is a Python library for constructing differentiable convex optimization layers in PyTorch, JAX, and TensorFlow using CVXPY. A convex optimization layer solves a parametrized convex optimization problem in the forward pass to produce a solution. It computes the derivative of the solution with respect to the …This paper presents a Python wrapper and extended functionality of the parallel topology optimization framework introduced by Aage et al. (Topology optimization using PETSc: an easy-to-use, fully parallel, open source topology optimization framework. Struct Multidiscip Optim 51(3):565–572, 2015). The Python interface, which simplifies …IBM CPLEX may be installed using pip install 'qiskit-optimization[cplex]' to enable the reading of LP files and the usage of the CplexOptimizer, wrapper for cplex.Cplex.CPLEX is a separate package and its support of Python versions is independent of Qiskit Optimization, where this CPLEX command will have no effect if there is no compatible version of … sys.flags.optimize gets set to 1. __debug__ is False. asserts don't get executed. In addition -OO has the following effect: sys.flags.optimize gets set to 2. doc strings are not available. To verify the effect for a different release of CPython, grep the source code for Py_OptimizeFlag. PyGAD - Python Genetic Algorithm!¶ PyGAD is an open-source Python library for building the genetic algorithm and optimizing machine learning algorithms. It works with Keras and PyTorch. PyGAD supports different types of crossover, mutation, and parent selection operators. PyGAD allows different types of problems to be optimized using the genetic …AFTER FINISHING THIS COURSE. Bayesian Machine Learning for Optimization in Python. Intermediate. 8h. Optimization theory seeks the best solution, which is pivotal for machine learning, cost-cutting in manufacturing, refining logistics, and boosting finance profits. This course provides a detailed description of different …Download a PDF of the paper titled Evolutionary Optimization of Model Merging Recipes, by Takuya Akiba and 4 other authors. We present a …4. No. The source code is compiled to bytecode only once, when the module is first loaded. The bytecode is what is interpreted at runtime. So even if you could put bytecode inline into your source, it would at most only affect the startup time of the program by reducing the amount of time Python spent converting the source code into bytecode.Learn how to solve optimization problems in Python using different methods: linear, integer, and constraint. See examples of how to import libraries, define v…Python Optimization Tips & Tricks. These tips and tricks for python code performance optimization lie within the realm of python. The following is the list of python performance tips. 1. Interning Strings for Efficiency. Interning a string is a technique for storing only one copy of each unique string.Sep 28, 2021 ... scipy.optimize.minimize can also handle some kinds of constraints. We examine how to minimize a function in Python where there are equality ...Python has become one of the most popular programming languages in recent years. Whether you are a beginner or an experienced developer, there are numerous online courses available...The Nelder-Mead optimization algorithm can be used in Python via the minimize () function. This function requires that the “ method ” argument be set to “ nelder-mead ” to use the Nelder-Mead algorithm. It takes the objective function to be minimized and an initial point for the search. 1. 2.Optlang is a Python package for solving mathematical optimization problems, i.e. maximizing or minimizing an objective function over a set of variables subject to a number of constraints. Optlang provides a common interface to a series of optimization tools, so different solver backends can be changed in a …Linear optimization problems with conditions requiring variables to be integers are called integer optimization problems. For the puzzle we are solving, thus, the correct model is: minimize y + z subject to: x + y + z = 32 2x + 4y + 8z = 80 x, y, z ≥ 0, integer. Below is a simple Python/SCIP program for solving it.Dec 31, 2016 · 1 Answer. Sorted by: 90. This flag enables Profile guided optimization (PGO) and Link Time Optimization (LTO). Both are expensive optimizations that slow down the build process but yield a significant speed boost (around 10-20% from what I remember reading). The discussion of what these exactly do is beyond my knowledge and probably too broad ... Nov 12, 2020 ... Title:tvopt: A Python Framework for Time-Varying Optimization ... Abstract:This paper introduces tvopt, a Python framework for prototyping and ...And run the optimization: results = skopt.forest_minimize(objective, SPACE, **HPO_PARAMS) That’s it. All the information you need, like the best parameters or scores for each iteration, are kept in the results object. Go here for an example of a full script with some additional bells and whistles.Newton’s method for optimization is a particular case of a descent method. With “ f′′ (xk ) ” being the derivative of the derivative of “ f” evaluated at iteration “ k”. Consider ...Optimization - statsmodels 0.14.1. Optimization ¶. statsmodels uses three types of algorithms for the estimation of the parameters of a model. Basic linear models such as WLS and OLS are directly estimated using appropriate linear algebra. RLM and GLM, use iteratively re-weighted least squares.Apr 11, 2023 ... Python processes can share .dll or .so memory but cannot share the memory used for python code. Only by using a single process can you avoid ...Are you looking to enhance your programming skills and boost your career prospects? Look no further. Free online Python certificate courses are the perfect solution for you. Python.... Apr 24, 2023 · Before diving into optimization techniques, it'Here are three strategies to accelerate Optimization Loop¶ Once we set our hyperparameters, we can then train and optimize our model with an optimization loop. Each iteration of the optimization loop is called an epoch. Each epoch consists of two main parts: The Train Loop - iterate over the training dataset and try to converge to optimal parameters. Python has become one of the most widely used programming languages in the world, and for good reason. It is versatile, easy to learn, and has a vast array of libraries and framewo... The capability of solving nonlinear least-squares problem with The Python ecosystem offers several comprehensive and powerful tools for linear programming. You can choose between simple …Python is a popular programming language known for its simplicity and versatility. Whether you’re a seasoned developer or just starting out, understanding the basics of Python is e... Oct 12, 2021 · Optimization refers to a procedure for finding the ...
Continue Reading