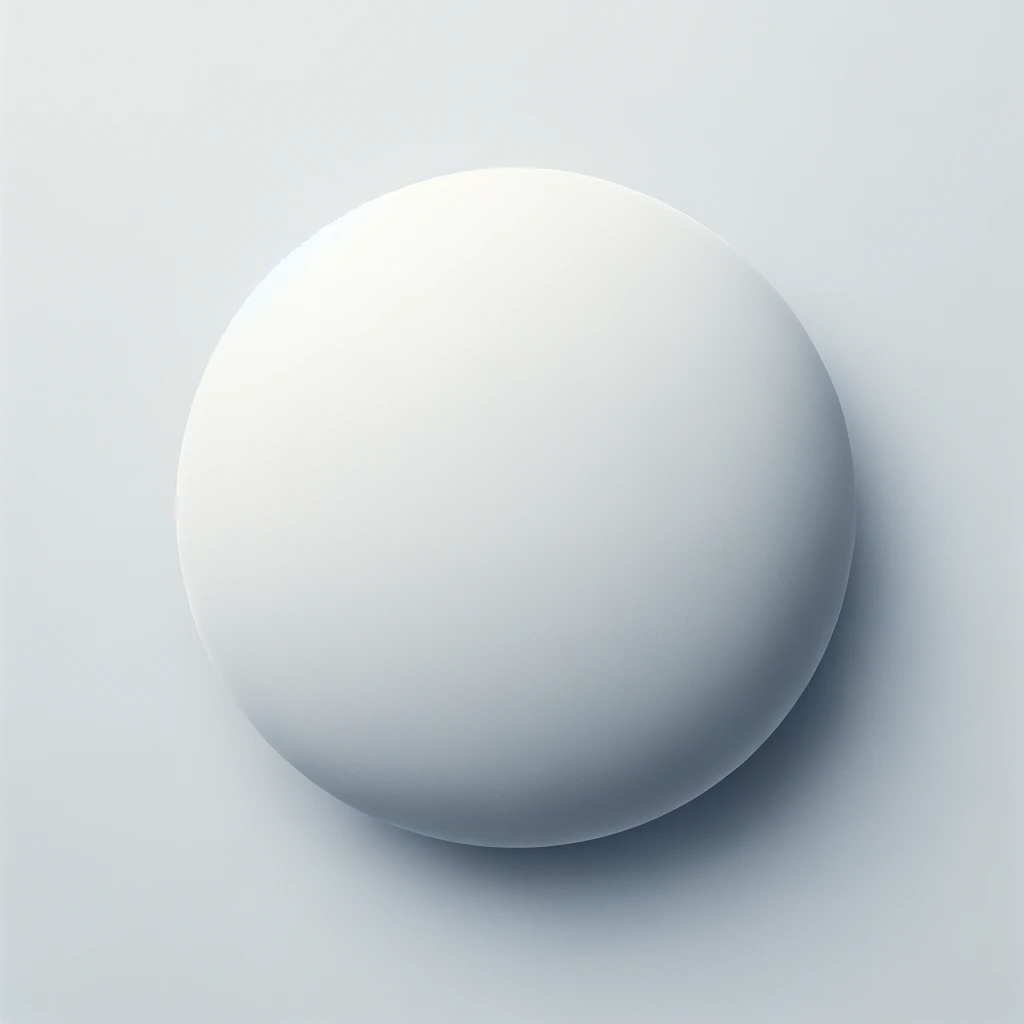
Algorithm. The bubble sort algorithm works as follows. Step 1) Get the total number of elements. Get the total number of items in the given list. Step 2) Determine the number of outer passes (n – 1) to be done. Its length is list minus one. Step 3) Perform inner passes (n – 1) times for outer pass 1.Python Lists. A Python list is a built-in data structure that holds a collection of items. To understand how lists work, let’s go straight to the example. We want to create a list that includes names of the US presidents elected in the last six presidential elections. List elements should be enclosed in square brackets [] andPython Examples: This Python programming examples page covers a wide range of basic concepts in the Python language, including list, strings, tuple, array, matrix, sets, and many more. Explore this Python program example page and …Dec 1, 2023 · More Example on List index() Method. We will cover different examples to find the index of element in list using Python, and explore different scenarios while using list index() method, such as: Python Tuple Data Type. Tuple is an ordered sequence of items same as a list. The only difference is that tuples are immutable. Tuples once created cannot be modified. In Python, we use the parentheses to store items of a tuple. For example, product = ('Xbox', 499.99) Here, product is a tuple with a string value Xbox and integer value 499.99.Dec 27, 2023 · Below are some examples which depict the use of list comprehensions rather than the traditional approach to iterate through iterable: Python List Comprehension using If-else. In the example, we are checking that from 0 to 7 if the number is even then insert Even Number to the list else insert Odd Number to the list. Jul 19, 2023 · Learn how to create, access, modify, sort, and use lists in Python. This tutorial covers the basics and advanced features of lists, such as slicing, copying, comprehensions, and functional tools. In the following example, we will define a list with different datatypes. Python Program. list1 = ["Apple", 54, 87.36, True] print(list1) Run Code Copy. Output. ['Apple', 54, 87.36, …When we find the target element, we calculate the negative index using the formula - (i+1) and break out of the loop. If the element is not found in the list, the index variable remains at its initial value of -1. Finally, we print the negative index. Python3. test_list = [5, 7, 8, 2, 3, 5, 1]2 Mar 2020 ... Python allows updating single or multiple elements in lists. The following are some examples depicting list update: Deleting Lists: Python ...In this exercise, you will need to add numbers and strings to the correct lists using the "append" list method. You must add the numbers 1,2, and 3 to the "numbers" list, and the words 'hello' and 'world' to the strings variable. You will also have to fill in the variable second_name with the second name in the names list, using the brackets ...From the project directory, follow steps to create the basic structure of the app: Open a new text file in your code editor. Add import statements, create the structure for the program, and include basic exception handling, as shown below. Save the new file as blob_quickstart.py in the blob-quickstart directory.You can use the insert () method to insert an item to a list at a specified index. Each item in a list has an index. The first item has an index of zero (0), the second has an index of one (1), and so on. Here's an example using the insert () method: myList = ['one', 'two', 'three'] A list, a type of sequence data, is used to store the collection of data. Tuples and Strings are two similar data formats for sequences. Lists written in Python are identical to dynamically scaled arrays defined in other languages, such as Array List in Java and Vector in C++. A list is a collection of items separated by commas and denoted by ... In Python, we use = operator to create a copy of an object. You may think that this creates a new object; it doesn't. It only creates a new variable that shares the reference of the original object. Let's take an example where we create a list named old_list and pass an object reference to new_list using = operator. Example 1: Copy using = operatorHi! This short tutorial will demonstrate what a list node is in the Python programming language. A list node is typically used in the context of linked list data structure, where each node contains a value and a reference to the next node in the list. The table of content is structured as follows: 1) Create Sample Linked List Structure.The for loop in Python is an iterating function. If you have a sequence object like a list, you can use the for loop to iterate over the items contained within the list. The functionality of the for loop isn’t very different from what you see in multiple other programming languages. Learn how to create, access, modify and perform operations on lists in Python. Lists are containers of values of different data types in contiguous blocks of memory. Python is a popular programming language known for its simplicity and versatility. Whether you’re a seasoned developer or just starting out, understanding the basics of Python is e...Python List with Examples – A Complete Python List Tutorial. What is Python List? Unlike C++ or Java, Python Programming Language doesn’t have arrays. To hold a …The list() returns a list object from an iterable passed as arguement with all elements.Python Program to Concatenate Two Lists . To understand this example, you should have the knowledge of the following Python programming topics:. Python List; Python List extend() Examples. 1. Sort elements in a list. Following is simple example program where we use sorted () built-in function to sort a list of numbers. We provide this list of numbers as iterable, and not provide key and reverse values as these are optional parameters. Python Program. nums = [2, 8, 1, 6, 3, 7, 4, 9] 3. Create a List of Names in Python. To create a list in Python, add comma-separated values between braces. For instance, here is a list of names in Python: names = ["Alice", "Bob", "Charlie"] 4. Merge Two Lists. Given two or more lists in Python, you may want to merge them into a single list. Python then finds itself stuck in a loop where neither import can complete first. Since app hasn't finished initializing (it's waiting to complete the import before it can …Jun 3, 2021 · How Lists Work in Python. It’s quite natural to write down items on a shopping list one below the other. For Python to recognize our list, we have to enclose all list items within square brackets ( [ ]), with the items separated by commas. Here’s an example where we create a list with 6 items that we’d like to buy. The files required in to-do list project are: tasks.txt – The text file where all our tasks will be stored. main.py – The python script file. Here are the steps you will need to execute to build this python project: Importing all the necessary libraries. Initializing the window and placing all the components in it.Python標準ライブラリのrandomモジュールのchoice(), sample(), choices()関数を使うと、リストやタプル、文字列などのシーケンスオブジェクトからランダムに要素を選択して取得(ランダムサンプリング)できる。. choice()は要素を一つ選択、sample(), choices()は複数の要素を選択する。We need mode ‘a’, for append. In the following example, we: Write to a file just like in the previous example using Python’s with open (). After that, we open the file again, this time with the append flag, and add some extra lines. Read back the file contents and print to screen so we can inspect the result.In a singly linked list, to delete a node, a pointer to the previous node is needed. To get this previous node, sometimes the list is traversed. In DLL, we can get the previous node using the previous pointer. Disadvantages of Doubly Linked List over the singly linked list: Every node of DLL Requires extra space for a previous pointer.Definition and Use of List insert() Method. List insert() method in Python is very useful to insert an element in a list. What makes it different from append() is that the list insert() function can add the value at any position in a list, whereas the append function is limited to adding values at the end.. It is used in editing lists with huge amount of data, as …grocery_list = ["apples", "bananas", "milk"] A Python list is a dynamic, mutable, ordered collection of elements enclosed within square brackets []. These …Learn how to create, access, modify, sort, and use lists in Python. This tutorial covers the basics and advanced features of lists, such as slicing, copying, … In this tutorial, you’ll learn how to: Reverse existing lists in place using .reverse () and other techniques. Create reversed copies of existing lists using reversed () and slicing. Use iteration, comprehensions, and recursion to create reversed lists. Iterate over your lists in reverse order. Aug 29, 2018 · sample () is an inbuilt function of random module in Python that returns a particular length list of items chosen from the sequence i.e. list, tuple, string or set. Used for random sampling without replacement. Syntax : random.sample (sequence, k) Parameters: sequence: Can be a list, tuple, string, or set. k: An Integer value, it specify the ... Are you looking to enhance your programming skills and boost your career prospects? Look no further. Free online Python certificate courses are the perfect solution for you. Python...Examining the first ten years of Stack Overflow questions, shows that Python is ascendant. Imagine you are trying to solve a problem at work and you get stuck. What do you do? Mayb...In Python, a list may contain items of different types. For example, # A list with 3 items of different types random_list = [5, 2.5, 'Hello'] A list may also contain another list as an …Learn how to define, manipulate, and use lists and tuples in Python, two of the most versatile and useful data types. See examples of ordered, mutable, and dynamic …If you are a Python programmer, it is quite likely that you have experience in shell scripting. It is not uncommon to face a task that seems trivial to solve with a shell command. ...Python List with Examples – A Complete Python List Tutorial. What is Python List? Unlike C++ or Java, Python Programming Language doesn’t have arrays. To hold a …Here's an example: # Joining list elements into a single string. all_fruits = ''.join(fruits) print(all_fruits) In this code snippet, we use an empty string '' as the …In Python, can use use the range() function to get a sequence of indices to loop through an iterable. You'll often use range() in conjunction with a for loop.. In this tutorial, you'll learn about the different ways in which you can use the range() function – with explicit start and stop indices, custom step size, and negative step size.. Let's get started.random.sample(range(len(mylist)), sample_size) generates a random sample of the indices of the original list. These indices then get sorted to preserve the ordering of elements in the original list. Finally, the list comprehension pulls out the actual elements from the original list, given the sampled indices. Share.Using List Comprehension. In this example, a list comprehension is used to succinctly generate the list odd_numbers by iterating through the elements of a 2D matrix. Only odd elements are included in the resulting list, providing a concise and readable alternative to the equivalent nested loop structure.Dec 21, 2023 · Here are some examples and use-cases of list append() function in Python. Append to list in Python using List.append() Method. List.append() method is used to add an element to the end of a list in Python or append a list in Python, modifying the list in place. For example: `my_list.append(5)` adds the element `5` to the end of the list `my_list`. For programmers, this is a blockbuster announcement in the world of data science. Hadley Wickham is the most important developer for the programming language R. Wes McKinney is amo...Example-2: Access string and integers inside list. Iterate over list items using for loop. Add elements to python list. Method-1: Append item to existing list using list.append (item) Method-2: Add element to a list using list.extend (iterable) Method-3: Add item at a certain position in a list.In this exercise, you will need to add numbers and strings to the correct lists using the "append" list method. You must add the numbers 1,2, and 3 to the "numbers" list, and the words 'hello' and 'world' to the strings variable. You will also have to fill in the variable second_name with the second name in the names list, using the brackets ...Two-dimensional lists (arrays) Theory. Steps. Problems. 1. Nested lists: processing and printing. In real-world Often tasks have to store rectangular data table. [say more on this!] Such tables are called matrices or two-dimensional arrays. In Python any table can be represented as a list of lists (a list, where each element is in turn a list).I have this big list of json elements composed of “categories” and “tags” for every category. (Example Below) I give this list of categories and tags to the model, …Python Lists. A Python list is a built-in data structure that holds a collection of items. To understand how lists work, let’s go straight to the example. We want to create a list that includes names of the US presidents elected in the last six presidential elections. List elements should be enclosed in square brackets [] andDec 6, 2023 · What is Linked List in Python. A linked list is a type of linear data structure similar to arrays. It is a collection of nodes that are linked with each other. A node contains two things first is data and second is a link that connects it with another node. Below is an example of a linked list with four nodes and each node contains character ... blue = colors[ 1 ] green = colors[ 2 ] Code language: Python (python) However, Python provides a better way to do this. It’s called sequence unpacking. Basically, you can assign elements of a list (and also a tuple) to multiple variables. For example: red, blue, green = colors Code language: Python (python) This statement assigns the first ...15 Mar 2021 ... I have to get, at a specific step of the loop, what is the list (a,b or c) a specific item comes from. For example, if the algorithm gives ...You say you want to list all directories within a bucket, but your code attempts to list all contents (not necessarily directories) within a number of buckets. These buckets probably do not exist (because they have illegal names). So when you run. bucket = s3.Bucket(name) bucket is probably null, and the subsequent list will fail.13 Jul 2023 ... get() function. You can access an item in the list by referring to its index number. Python list index starts from 0. The following are examples ... The Python list() constructor returns a list in Python. In this tutorial, we will learn to use list() in detail with the help of examples. Courses Tutorials Examples . . W3Schools offers free online tutorials, referencTry it out! This tutorial provides a basic Two-dimensional lists (arrays) Theory. Steps. Problems. 1. Nested lists: processing and printing. In real-world Often tasks have to store rectangular data table. [say more on this!] Such tables are called matrices or two-dimensional arrays. In Python any table can be represented as a list of lists (a list, where each element is in turn a list).How Lists Work in Python. It’s quite natural to write down items on a shopping list one below the other. For Python to recognize our list, we have to enclose all list items within square brackets ( [ ]), with the items separated by commas. Here’s an example where we create a list with 6 items that we’d like to buy. 30 Jan 2023 ... List in python, Python lists, Pyt Are you an intermediate programmer looking to enhance your skills in Python? Look no further. In today’s fast-paced world, staying ahead of the curve is crucial, and one way to do ... Sep 5, 2023 · Python's *for* and *in* constructs are e...
Continue Reading