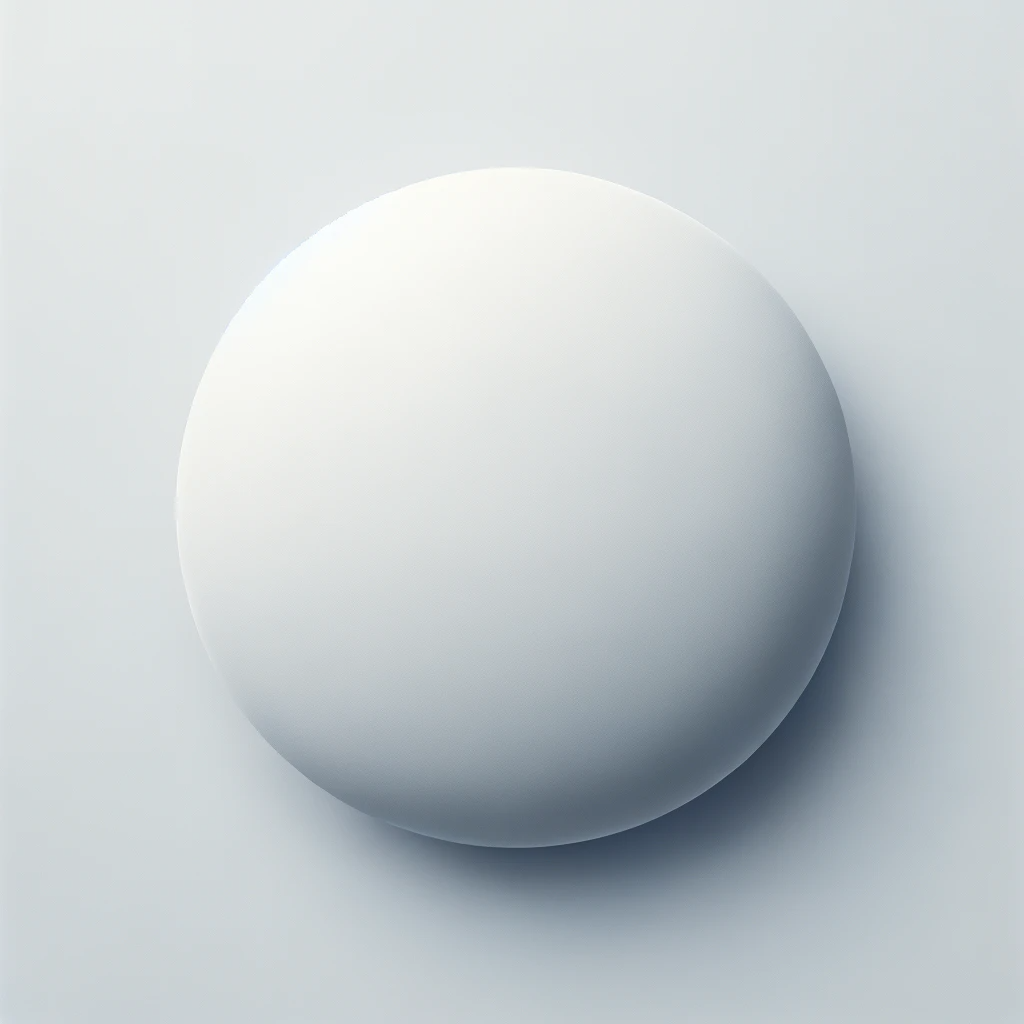
Append to the end of a string using the f-string. f-string is a new string formatting available on Python 3.6 or above. We can use an f-string to append a value to the end of a string. Example: # String. my_str = "Hello" # Append to the end of string using f-string print(f"{my_str} Python") Output: Hello Python.6.startswith() and endswith(): Checking the Start and End of a String. The startswith() method checks whether a string starts with a specified substring, while the endswith() method checks whether ...9.5. String Methods¶ ; tess.right(90) when we wanted the turtle object ; tess to perform the ; right method to turn to the right 90 degrees. The “dot notation” is ...pandas.Series.str. #. Series.str() [source] #. Vectorized string functions for Series and Index. NAs stay NA unless handled otherwise by a particular method. Patterned after Python’s string methods, with some inspiration from R’s stringr package.W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. Python has a set of built-in methods that you can use on dictionaries. Method. Description. clear () Removes all the elements from the dictionary. copy () Returns a copy of the dictionary. fromkeys () Returns a dictionary with the specified keys and value. The strptime () class method takes two arguments: string (that be converted to datetime) format code. Based on the string and format code used, the method returns its equivalent datetime object. In the above example: Here, %d - Represents the day of the month. Example: 01, 02, ..., 31. %B - Month's name in full.Strings are an essential data type in Python that are used in nearly every application. In this article we learn about the most essential built-in string methods. With this resource …The in operator in Python (for list, string, dictionary, etc.) Forward/backward match: startswith(), endswith() For forward matching, use the string method startswith(), which checks if a string begins with the specified string. Built-in Types - str.startswith() — Python 3.11.3 documentation W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.Collections of text in Python are called strings. Special functions called string methods are used to manipulate strings. There are string methods for changing a string from lowercase to uppercase, removing …"The mother must not be (seen to) cut corners or avoid pain." Pain-free childbirth already had a bad name in Japan, and it could get worse. The Japanese government is looking into ...May 17, 2023 ... Python Programming: String Methods in Python (Part 5) Topics discussed: 1. upper() Method for Strings in Python. 2. lower() Method for ...Checks if all characters in string are digits or integers. str.isidentifier() Checks if the string is a valid variable name. str.isnumeric() Checks if all characters in string are numeric. str.isspace() Checks if all characters in string are blank spaces. str.istitle() Checks if all words in the string start with a capital letter. str.isupper()Python has the useful string methods find() and replace() that help us perform these string processing tasks. In this tutorial, we'll learn about these two string methods with example code. Immutability of Python Strings. Strings in Python are immutable. Therefore, we cannot modify strings in place. Strings are Python iterables that follow zero-indexing.Collections of text in Python are called strings. Special functions called string methods are used to manipulate strings. There are string methods for changing a string from lowercase to uppercase, removing … W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. Built-in Types - str.format () — Python 3.11.3 documentation. In the string that calls the format () method, the {} is known as the replacement field, which is substituted with the format () method's argument. The format specification can be written after : in {}. Here is the equivalent code using the format () method, corresponding to the ...Mar 9, 2018 · format (format_string, /, *args, **kwargs) ¶. The primary API method. It takes a format string and an arbitrary set of positional and keyword arguments. It is just a wrapper that calls vformat (). Changed in version 3.7: A format string argument is now positional-only. vformat (format_string, args, kwargs) ¶. Learn about the most popular string methods in Python, including str.capitalize(), str.upper(), str.lower(), str.split(), str.replace(), str.join(), str.strip(), and str.find(). See examples of how to use these methods in real coding tasks and get a quick overview of their functionality with our concise code-based summary2 days ago · Built-in Types. ¶. The following sections describe the standard types that are built into the interpreter. The principal built-in types are numerics, sequences, mappings, classes, instances and exceptions. Some collection classes are mutable. The methods that add, subtract, or rearrange their members in place, and don’t return a specific ... Python Overview Python Built-in Functions Python String Methods Python List Methods Python Dictionary Methods Python Tuple Methods Python Set Methods Python File Methods Python Keywords Python Exceptions Python Glossary Module Reference Random Module Requests Module Statistics Module Math Module cMath Module Python How To Remove List …The Python str class has many useful features that can help you out when you’re processing text or strings in your code. However, in some situations, all these great features may not be enough for you. You may need to create custom string-like classes.To do this in Python, you can inherit from the built-in str class directly or subclass …Go ahead and admit it: you hate weeds. They’re pervasive and never seem to go away. You can win your battle with weeds when you have the right tools at your fingertips. A quality s... These methods help you deal with string cases effectively including lowercase, uppercase, titlecase, and casefold. Method. Description. lower () Return a copy of a string in lowercase. upper () Return a copy of a string in uppercase. title () Return a copy of a string in the titlecase. The escape character allows you to use double quotes when you normally would not be allowed: txt = "We are the so-called \"Vikings\" from the north." Try it Yourself ». Other escape characters used in Python: Code. Result. Try it. \'. Single Quote.Stringing a new basketball net typically involves stretching the net’s nylon loops around the metal hooks on the rim of the basketball hoop. If the current net on the hoop is old o...Jul 19, 2005 ... The proper way is to extend the string type by subclassing it: class S(str): def my_method(self): ... Then you can do "S('anystring').my_method .... How to delete files and folders in Python ; 31 essential String methods in Python you should know 31 essential String methods in Python you should know On this page . 1. Slicing ; 2. strip() 3./4. lstrip() and rstrip() strip() with character ; strip() with combination of characters ; 5./6. removeprefix() and removesuffix() 7. replace() 8. re ... Using Python String Methods. Lab. A Cloud Guru. Using Python String Methods. Strings are the primary way that we interact with non-numerical data in …6.startswith() and endswith(): Checking the Start and End of a String. The startswith() method checks whether a string starts with a specified substring, while the endswith() method checks whether ...Built-In String Methods: Overview. Strings and Character Data in Python Christopher Bailey 02:06. Mark as Completed. Supporting Material. Contents. Transcript. Discussion. …Join Sets element into a String using join() method. In this example, we are using a Python set to join the string. ... File "Solution.py", line 4, in <module> string = '_'.join(dic) TypeError: sequence item 0: expected string, int found Joining a list of Strings with a Custom Separator using Join() Python string is the collection of the characters surrounded by single quotes, double quotes, or triple quotes. The computer does not understand the characters; internally, it stores manipulated character as the combination of the 0's and 1's. Each character is encoded in the ASCII or Unicode character. So we can say that Python strings are ... We would like to show you a description here but the site won’t allow us.Jun 15, 2012 at 13:45. Add a comment. 3. You can use str.split ( [sep [, maxsplit]]) Return a list of the words in the string, using sep as the delimiter string. If maxsplit is given, at most maxsplit splits are done (thus, the list will have at most maxsplit+1 elements).VIN stands for vehicle identification number, and it’s a 17-character string of letters and numbers that tell you about the vehicle’s specifications and its manufacturing history. ...Strings are a fundamental data type in Python and play a critical role in many data processing tasks, such as text analysis, natural language processing, and …Public methods (including the __init__ constructor) should also have docstrings. A package may be documented in the module docstring of the __init__.py file in the package directory. String literals occurring elsewhere in … These methods help you deal with string cases effectively including lowercase, uppercase, titlecase, and casefold. Method. Description. lower () Return a copy of a string in lowercase. upper () Return a copy of a string in uppercase. title () Return a copy of a string in the titlecase. 17./18./19. isalpha(), isnumeric(), isalnum()¶ isalpha(): Return True if all characters in the string are alphabetic and there is at least one character, False otherwise.isnumeric(): Return True if all characters in the string are numeric characters, and there is at least one character, False otherwise.isalnum(): Return True if all characters in the string are …YouTube TV is giving subscribers free access to the EPIX channel through April 25, throwing a lifeline to users running out of stuff to watch on their self-quarantine backlog. YouT...The syntax of lower() method is: string.lower() lower() Parameters() lower() method doesn't take any parameters. lower() Return value. lower() method returns the lowercase string from the given string. It converts all uppercase characters to lowercase. Definition and Usage. The format () method formats the specified value (s) and insert them inside the string's placeholder. The placeholder is defined using curly brackets: {}. Read more about the placeholders in the Placeholder section below. The format () method returns the formatted string. Repeat String. In the above example, you can observe that the string "PFB "is repeated 5 times when we multiply it by 5.. Replace Substring in a String in Python. You can replace a substring with another substring using the replace() method in Python. The replace() method, when invoked on a string, takes the substring to replaced as its first …The startswith() method returns True if the string starts with the specified value, otherwise False. Syntax. string.startswith(value, start, end) Parameter Values. Parameter Description; value: Required. The value to check if the string starts with: start: Optional.Definition and Usage. The rfind () method finds the last occurrence of the specified value. The rfind () method returns -1 if the value is not found. The rfind () method is almost the same as the rindex () method. See example below.The Python str class has many useful features that can help you out when you’re processing text or strings in your code. However, in some situations, all these great features may not be enough for you. You may need to create custom string-like classes.To do this in Python, you can inherit from the built-in str class directly or subclass …You don’t have to dig a big hole in your yard to cut a piece of plastic PVC pipe that’s buried in the ground. Watch this video to find out how to cut PVC pipe using nothing more th...The built-in str class allows you to create strings in Python. Strings are sequences of characters that you’ll use in many situations, especially when working with textual data. From time to time, the standard functionalities of Python’s str may be insufficient to fulfill your needs. So, you may want to create custom string-like classes that solve your specific problem. ... For example, if …"Many people feel like they're on a journey to see what's beyond everyday life. Physics says you don't have to look far to find that. It's right around the corner." Physics is the ...As with any dairy-based product, string cheese should be refrigerated until it is ready to be eaten. String cheese is safe to eat for up to 2 hours before it should be refrigerated...The built-in str class allows you to create strings in Python. Strings are sequences of characters that you’ll use in many situations, especially when working with textual data. From time to time, the standard functionalities of Python’s str may be insufficient to fulfill your needs. So, you may want to create custom string-like classes that solve your specific problem. ... For example, if …Join Sets element into a String using join() method. In this example, we are using a Python set to join the string. ... File "Solution.py", line 4, in <module> string = '_'.join(dic) TypeError: sequence item 0: expected string, int found Joining a list of Strings with a Custom Separator using Join()The Python String len () method is used to retrieve the length of the string. A string is a collection of characters and the length of a string is the number of characters (Unicode values) in it. The len () method determines how many characters are there in the string including punctuation, space, and all type of special characters.Python offers many ways to substring a string. This is often called "slicing". Here is the syntax: string[start:end:step] Where, start: The starting index of the substring. The character at this index is included in the substring. If start is not included, it is assumed to equal to 0. end: The terminating index of the substring.In this article, we have covered about Python string len() function and discussed its working. len() function is used to find the length of a string. It is a very common yet important function of string methods. Read More String Methods. Also Read: Find length of a string in python (6 ways) Find the length of a String; Python len() FunctionSometimes the apron strings can be tied a little too tightly. Read about how to cut the apron strings at TLC Weddings. Advertisement As the mother of two handsome, brilliant and ot...Dec 27, 2022 ... Find and Replace · encode(): it encodes the string using a specified encoding scheme. · find(): it returns the lowest index of the specified ...Python String Methods: Overview. Python String Methods that Return a Modified Version of a String. Python String Methods for Joining and Splitting Strings. …Method: In Python, we can use the function split() to split a string and join() to join a string. the split() method in Python split a string into a list of strings after breaking the given string by the specified separator. Python String join() method is a string method and returns a string in which the elements of the sequence have been …Built-In String Methods: Overview. Strings and Character Data in Python Christopher Bailey 02:06. Mark as Completed. Supporting Material. Contents. Transcript. Discussion. …Watch this video to find out about the EGO Power+ cordless string trimmer powered by a 56-volt, lithium-ion battery for increased performance and run time. Expert Advice On Improvi... How to Confirm That a Python String Contains Another String. If you need to check whether a string contains a substring, use Python’s membership operator in. In Python, this is the recommended way to confirm the existence of a substring in a string: Python. >>> raw_file_content = """Hi there and welcome. ... Python has a set of built-in methods that you can use on strings. Note: All string methods returns new values. They do not change the original string. Note: All string methods returns new values. They do not change the original string. Learn more about …Python string methods offer a variety of ways to inspect and categorize string content. Among these, the isalpha(), isdigit(), isnumeric(), and isalnum() methods are commonly used for checking the character composition of strings. First of all, let's discuss the isalpha() method. You can use it to check whether all characters in a string are alphabetic (i.e., letters … W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. Python Overview Python Built-in Functions Python String Methods Python List Methods Python Dictionary Methods Python Tuple Methods Python Set Methods Python File Methods Python Keywords Python Exceptions Python Glossary ... The startswith() method returns True if the string starts with the specified value, otherwise False. Syntax. string.startswith(value, …The ljust() method will left align the string, using a specified character (space is default) as the fill character. Syntax. string.ljust(length, character) Parameter Values. Parameter Description; length: Required. The length of the returned string: character: Optional. A character to fill the missing space (to the right of the string).Dec 19, 2021 · Strings are an essential data type in Python that are used in nearly every application. In this tutorial we learn about the 31 most important built-in string... . Strings are a fundamental data type in Python and W3Schools offers free online tutorials, refere Sure you can hang clothes on the shower rod or be content with a simple drying rack in the laundry room. This DIY indoor clothes line, however, makes excellent use of a small space... Strings in Python are represented using the built-in str type/class. File "main.py", line 1. my_string = 'It's a lovely day!' ^ SyntaxError: invalid syntax. ... Python has a number of built-in string methods that manipulate strings. However, when these methods are called, the original string will not be changed, so any modifications will need to be saved to a new variable. A few useful built-in string methods ...2 days ago · Built-in Types. ¶. The following sections describe the standard types that are built into the interpreter. The principal built-in types are numerics, sequences, mappings, classes, instances and exceptions. Some collection classes are mutable. The methods that add, subtract, or rearrange their members in place, and don’t return a specific ... Original string: roses are red After using capitalzie: Roses are...
Continue Reading